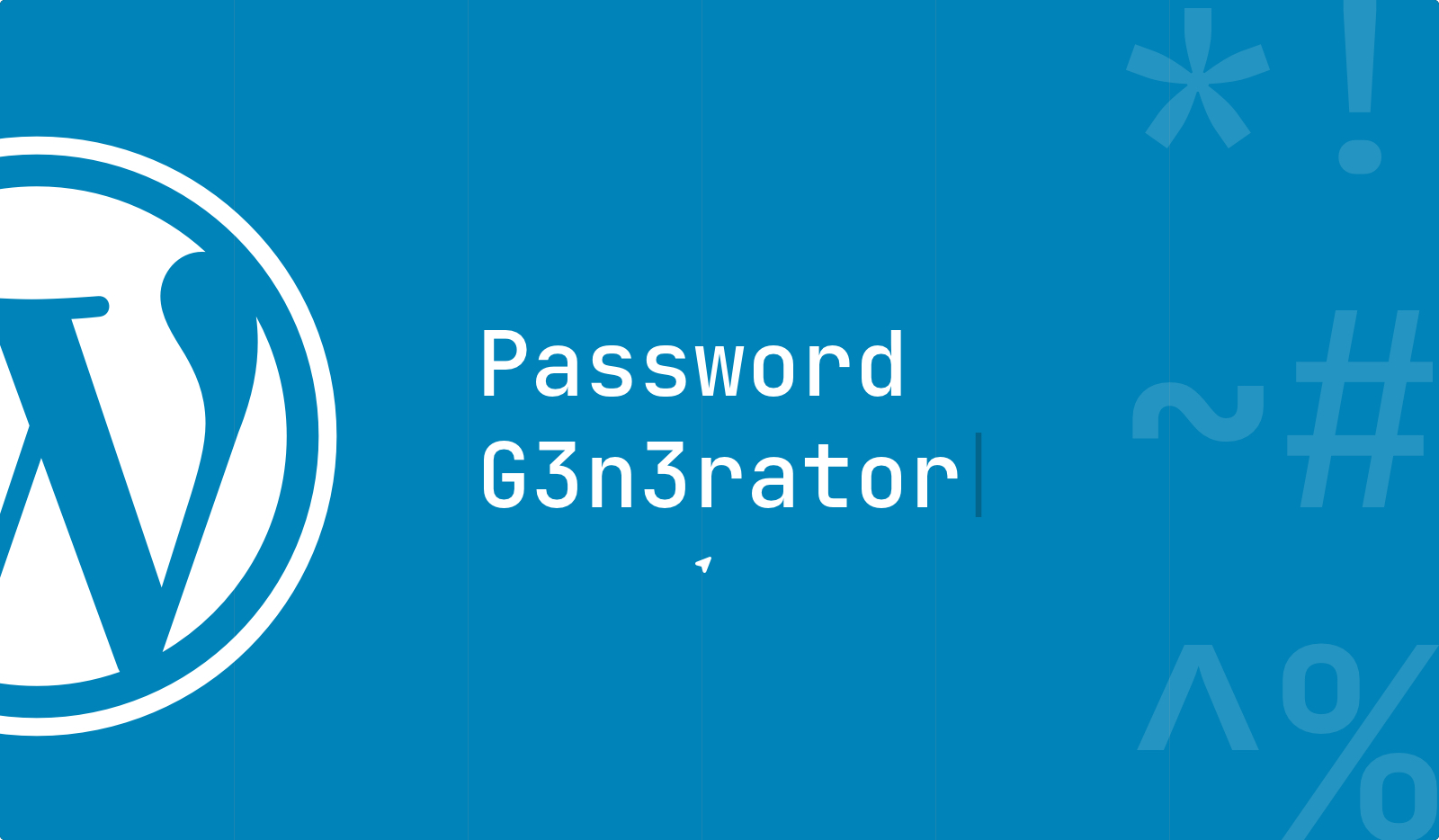
Have you forgotten your password again? No worries I forget mine as well from time to time. If you have forgotten your password, you can always use the Forgot your password?
option to generate a new password. It only becomes difficult if you no longer have access to the e-mail address of the account, or if the function is not working – as a result of a bug or outdated plugin.
Resetting passwords for your site can be a very tedious task, especially if you manage multiple websites. Logging into PHPMyAdmin or via the command line is probably the least enjoyable part of the process. While resetting your password is not too difficult, it often consists of repetitive tasks and requires attention to detail.
What we will do
In this tutorial, we will establish a way to use Javascript to create a WordPress passwords with Polypad.
Here’s what we’re going to do:
- Download prerequisites (Polypad, Scriptist)
- Convert NPM Library for helper
- Create our script code
- Run scripts using Polypad
At the end of this article, you will be able to generate WordPress passwords on the fly for your websites with minimal manual effort.
When we are done the finished program will look something like this base from Polypad:
Sounds simple, and it is, so let’s get started.
Prerequisites
In order to follow along this tutorial you will need to download the following software programs:
Polypad
Polypad is a cross-platform, scriptable textpad that allows developers to write scripts using javascript to transform, generate, or analyze text
Scriptist
If you would like to simply install the script without writing any code you can do so using scriptist – a script package manager for Polypad.
Once you have installed scriptist you can simply run:
scriptist install wpp
Phpass
To avoid reinventing the wheel, we will utilize a NPM package to help us generate passwords on the fly. We will utilize the node-phpass
library for helping us generate our wordpress password.
Note: Polypad does not allow direct npm module installations
Download the Phpass library from Github. We will make modifications to this libary to allow us to use within Polypad.
Setup Directory Structure
Once Polypad is installed and opened, navigate to Scripts -> Browse Scripts
menu option. This will open the scripts directory. We will use this directory to write our scripts and add external libraries.
The default directory structure should look something like so:
scripts
├── lib
Create a file within the scripts directory called WPasswordGenerator.js
. Now open the Phpass directory and copy the contents inside of the lib directory into the scripts/lib
directory. You directory structure should now look like this.
scripts
├── lib
│ ├── bcrypt.js
│ ├── passwordhash.js
│ └── utils.js
└── WPasswordGenerator.js
Now that our directory structure is setup. We will begin modifying the libary files. Open the bcrypt.js
file and change the require statement.
var utils = require('./utils');
to
var utils = require('@polypad/utils');
Repeat the process for the the passwordhash.js
file. We do not need to edit the utils.js file.
var BCrypt = require('@polypad/bcrypt').BCrypt;
var utils = require('@polypad/utils');
Creating our script
Now that our library is ready to go, we can finally create our script. Open the WPasswordGenerator.js
file and create the basic script structure.
/*
name: WordPress Password Generator
description: Generate password for WP
author: Marcell
tags: wordpress, password, generator
*/
// Main function to accept text input
function main(input) {
}
Now lets include our library so that we can access it within our script. Place the code below, under the script header
const PasswordHash = require('@polypad/passwordhash').PasswordHash;
Finally we will write our function body, which includes the password generator library and enables us to use it within our script.
/*
name: WordPress Password Generator
description: Generate password for WP
author: Marcell
tags: wordpress, password, generator
*/
const PasswordHash = require('@polypad/passwordhash').PasswordHash;
// Main function to accept text input
function main(input) {
var passwordHash = new PasswordHash();
var hash = passwordHash.hashPassword(input.text);
input.text = hash;
}
Now open Polypad and from the menu select Scripts -> Reload Scripts
. Now type a password string and press CTRL + P
and select the script. You should now be able to generate wordpress passwords on the fly.